Working with GPS in Android apps
22.09.2011 14:36 · Notes · android, howto
Most Android devices can determine their position with some accuracy. This is achieved by using the Global Positioning System (GPS), triangulation from mobile network base stations, or by using open (public) hotspots. To use these features, we need the android.location
package.
There are several classes in this package. The most important ones are:
LocationManager
— provides access to the system location services, i.e., GPS and others. It also allows you to select the service that best meets your criteria (power consumption, positioning accuracy, etc.)LocationProvider
is an abstract class from which all providers of geographic location information are derived. The provider periodically provides information about changes in geographic coordinates of the deviceLocationListener
— used to receive notifications from theLocationProvider
when coordinates change
Other classes that are worth mentioning are Location
(geographical location of the device at a given point in time) and Criteria
(criteria for selecting a provider).
In this post, I will focus on getting coordinates from the GPS_PROVIDER
provider.
To allow the application to receive data about the geographical position of the device, the AndroidManifets.xml
file must contain the following lines
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION">
<uses-feature android:name="android.hardware.location.gps">
I will not describe the process of creating the Android application in detail here but only give the code that implements “listening” to GPS.
package com.example.anddemo;
import android.app.Activity;
import android.os.Bundle;
import android.content.Context;
import android.location.Location;
import android.location.LocationListener;
import android.location.LocationManager;
import android.location.LocationProvider;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
public class AppActivity extends Activity {
private EditText etLatitude;
private EditText etLongitude;
private LocationManager mLocationManager;
private LocationListener mLocationListener;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// fields to show coordinates
etLatitude = (EditText) findViewById(R.id.txtLat);
etLongitude = (EditText) findViewById(R.id.txtLon);
// LocationManager instance
mLocationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE);
// try to get last known position
Location currentLocation = mLocationManager.getLastKnownLocation(LocationManager.GPS_PROVIDER);
// if it is valid, then fill fields with the values
if (currentLocation != null) {
float lat = (float) (currentLocation.getLatitude());
float lon = (float) (currentLocation.getLongitude());
etLatitude.setText(Float.toString(lat));
etLongitude.setText(Float.toString(lon));
} else {
etLatitude.setText("No location info");
etLongitude.setText("No location info");
}
// create listener, to "listen" for coordinate changes
mLocationListener = new LocationListener() {
public void onStatusChanged(String provider, int status,
Bundle extras) {
switch (status) {
case LocationProvider.OUT_OF_SERVICE:
Log.v("GPS demo",
"Status changed: " + provider + " provider"
+ " out of service");
break;
case LocationProvider.TEMPORARILY_UNAVAILABLE:
Log.v("GPS demo",
"Status changed: " + provider + " provider"
+ " temporarily unavailable");
break;
case LocationProvider.AVAILABLE:
Log.v("GPS demo",
"Status changed: " + provider + " provider"
+ " available");
break;
}
}
public void onProviderEnabled(String provider) {
Log.v("GPS demo", "Enabled new provider: " + provider);
}
public void onProviderDisabled(String provider) {
Log.v("GPS demo", "Disabled provider: " + provider);
}
public void onLocationChanged(Location location) {
Log.v("GPS demo", "Location changed");
float lat = (float) (location.getLatitude());
float lon = (float) (location.getLongitude());
etLatitude.setText(Float.toString(lat));
etLongitude.setText(Float.toString(lon));
}
};
}
/* at application start connect listener with manager and request updates
* with maximum frequency (in the real-world application frequence of request
* should be set to some reasonable value)
*/
protected void onResume() {
super.onResume();
mLocationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER,
0, 0, mLocationListener);
}
// stop tracking position changes when the application is paused
protected void onPause() {
super.onPause();
mLocationManager.removeUpdates(mLocationListener);
}
}
You can test it either on a real device or using an emulator. Make sure that the GPS module is active before running the application. If you are using an emulator, you can change the coordinates in DDMS
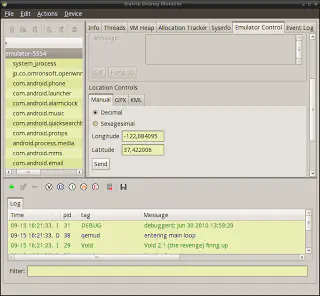
or via telnet
# connect to the device (5554 is your emulator ID)
telnet localhost 5554
# send coordinates (longitude latitude)
geo fix 48 51